Intelligent Text Parsing – Day 2: Building the AI Workflow
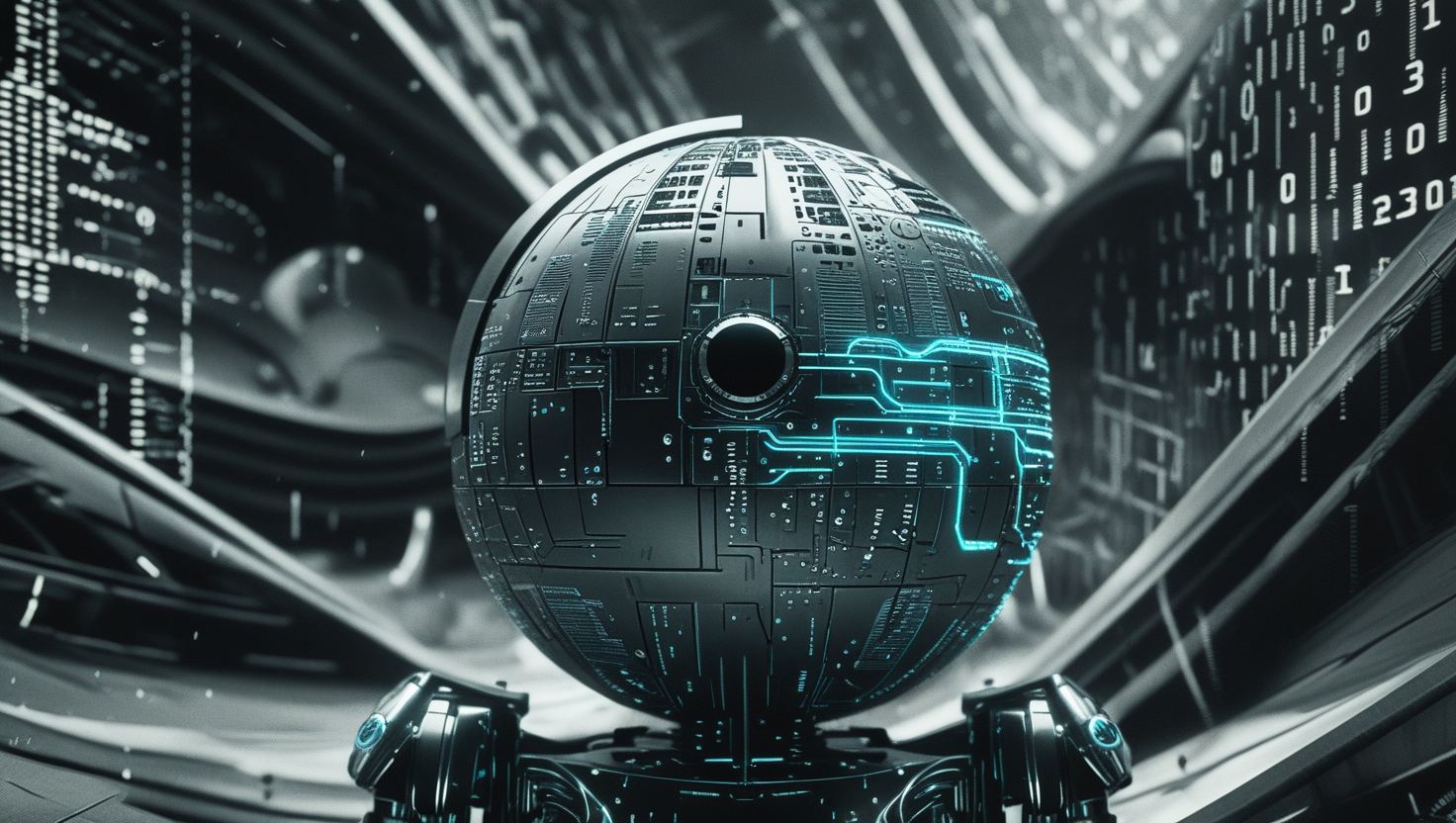
Welcome to Day 2 of our NeurochainAI No-Code Workshop!
Today, you’ll learn how to create a powerful automation that reads natural language messages, extracts specific information using AI, and sends back structured replies - all inside n8n.
Let’s build it step-by-step!
How the Workflow Works
Here’s the logic we’ll build:
- A user sends a message on Telegram.
- We define which fields we want to extract from that message.
- A small Code node generates the correct prompt for the AI.
- A HTTP Request sends the prompt to NeurochainAI IaaS.
- The AI returns only the structured info we asked for.
- A Telegram node replies with the result.
- (Optional) A Supabase node stores the info in a database.
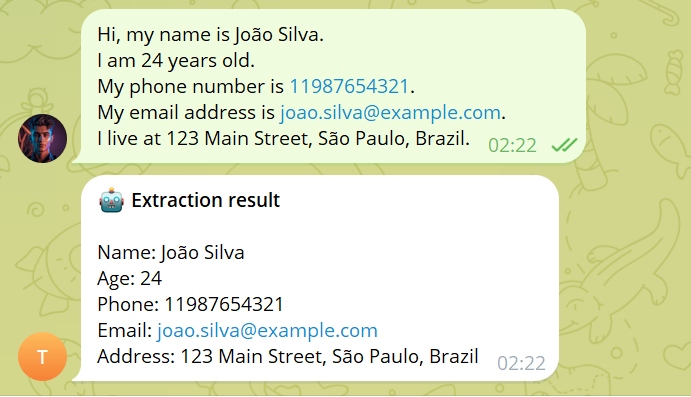
Step 1 – Telegram Trigger
Add a Telegram Trigger node to start the workflow when a user sends a message.
No extra configuration is needed here if your bot is already connected (check Day 1 if not).

Step 2 – Define What You Want to Extract
Next, add the node called Edit Fields (Set).
In this node, you’ll add Field Name and Value pairs.
- Field Name → what you want to extract (e.g., Phone, Name, Age)
- Value → a clear description of how the AI should extract it
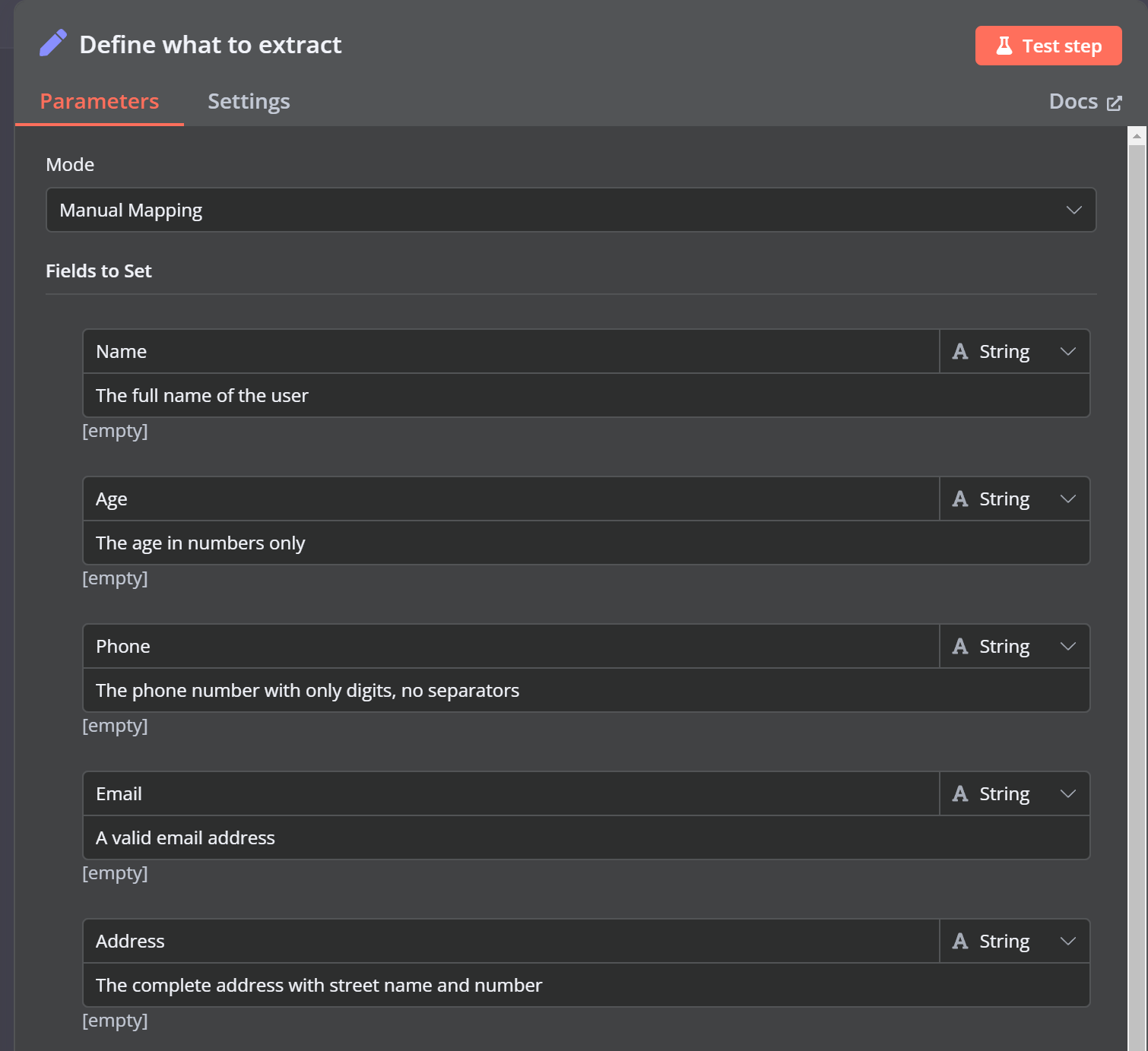
Example:
Name
The full name of the user
Age
The age in numbers only
Phone
The phone number with only digits, no separators
A valid email address
Address
The complete address with street name and number
Add as many fields as you want — the AI will extract them all in one shot.
Step 3 – Code Node (Optional, but Super Useful)
While this step is optional, we created a Code Node to make your life way easier.
Instead of writing or updating the AI prompt manually every time you change the fields in the Set node, this code dynamically generates everything for you.
That means:
If you change what you want to extract, you only need to update the Set node — nothing else.
Here’s the code to paste inside:
const fields = $json;
const instructions = [];
const fieldList = [];
for (const [key, value] of Object.entries(fields)) {
instructions.push(`• ${key}: ${value.trim()}`);
fieldList.push(key);
}
const telegramNode = "Telegram Trigger";
const telegramData = $node[telegramNode]?.json || {};
const messageText = telegramData.message?.text || "";
const chatId = telegramData.message?.chat?.id || "";
return [
{
json: {
extractionInstructions: `Please extract the following information:\n\n${instructions.join('\n')}`,
fieldList: fieldList.join(', '),
message: messageText,
chatId: chatId
}
}
];
This makes everything dynamic — no need to update the prompt manually every time.
Step 4 – Call the AI (HTTP Request Node)
Now add an HTTP Request node to connect with NeurochainAI IaaS.
Basic Settings:
- Method: POST
- URL: https://ncmb.neurochain.io/tasks/message
Headers:
- Authorization: Bearer YOUR-API-KEY-HERE
- Content-Type: application/json
- Body Content Type: JSON
- Specify Body: Use Fields Below
Add the following fields under the "Body Parameters":
Name
Value
model
Meta-Llama-3.1-8B-Instruct-Q6_K_L.gguf
prompt
(Paste the prompt below)
max_tokens
1024
temperature
0.3
top_p
0.95
frequency_penalty
0
presence_penalty
1.1
Prompt Field (copy exactly this into the prompt value):
You are an expert information extractor.\n\nYour task is to analyze the message below and return **only** the requested information, using the exact field names provided.\n\nThe output must follow this exact format:\nFieldName: value\nFieldName: value\n...\n\nEach result on a new line. Do not return any explanations, introductions, or additional formatting.\n\nIf any value is missing from the message, return the field name followed by an empty value (e.g., Age:).\n\n### Message to analyze:\n{{ $json.message }}\n\n### Fields to extract:\n{{ $json.extractionInstructions }}\n\n**Field names (must match exactly):**\n{{ $json.fieldList }}\n\n**Return only the extracted information.**

Step 5 – Respond on Telegram
Now add a Telegram node to send the result back to the user.
Text:
🤖 *Extraction result*
{{ $json.choices[0].text }}
Chat ID:
{{ $('Code').item.json.chatId }}
This makes the bot reply with the structured info you requested.
Step 6 – (Optional) Save to Supabase
You can add a Supabase node to store the extracted fields into a table.
Map each field (e.g., Name, Age, Phone) to the columns you created in your Supabase project.
This is great if you want to create dashboards or keep a log of submissions.
Bonus: Import the Full Template
We’ve made it easy for you:
👉 Download the Full Template JSON and import it into your n8n editor.

That’s it - Your AI Parser is Ready!
Congratulations! You’ve just built a fully functional AI-powered text parser using NeurochainAI IaaS and n8n - all without writing complex code.
Your automation is now capable of:
- Receiving natural messages from Telegram
- Extracting exactly the fields you define
- Replying instantly with structured data
- (Optionally) Saving everything to a database
-
-
You now have a solid, customizable foundation ready to be used in real-world scenarios.
Tomorrow in Day 3, we’ll do a quick polish and wrap-up. See you there!